Java Final vs Finally vs Finalize
Hello there, fellow Java enthusiasts! Today, we’re going to dive into a topic that often causes confusion among Java learners: the difference between final
, finally
, and finalize
. These three terms might sound similar, but they serve entirely different purposes in Java. So, buckle up, and let’s demystify these concepts together!
Understanding Java’s Final Keyword
The final
keyword in Java is used to restrict users. When you declare a variable as final, its value can’t be modified, it becomes a constant. Let’s see an example:
final int MAX_SPEED = 100;
JavaIn this case, the MAX_SPEED
variable is declared as final, meaning its value is constant and cannot be changed later in the code.
Similarly, you can use the final
keyword with methods and classes. A final
method cannot be overridden by any subclass, and a final
class cannot be subclassed. This is particularly useful when you want to make sure that your method implementation remains constant and that no other class can extend your class.
Understanding Java’s Finally Block
Moving on to finally
, it’s a block of code used in exception handling. The finally
block always executes, regardless of whether an exception is thrown or not. It’s usually used for cleanup code. Here’s an example:
try {
// risky code
} catch (Exception e) {
// handling exception
} finally {
// cleanup code
}
JavaIn this example, no matter what happens in the try
and catch
blocks, the finally
block will always execute, ensuring that the cleanup code runs.
Understanding Java’s Finalize Method
Last but not least, we have finalize
. It’s a special method in Java that the garbage collector calls before collecting objects that are eligible for garbage collection. It’s usually overridden to clean up system resources, like closing files or network connections. Here’s an example:
protected void finalize() {
// cleanup code
}
JavaIn this example, the finalize
method is overridden to include cleanup code that runs right before the object is garbage collected.
Understanding Java’s Final, Finally and Finalize with Diagram
The diagram illustrates the basic concepts of final
, finally
, and finalize
in Java.
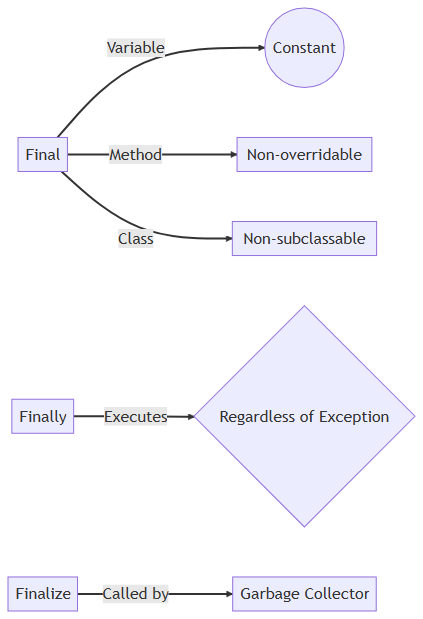
Final
is a keyword used to apply restrictions. When applied to a variable, it becomes a constant. When applied to a method, it becomes non-overridable, meaning it cannot be overridden in a subclass. When applied to a class, it becomes non-subclassable, meaning it cannot be extended by another class.Finally
is a block of code that always executes, regardless of whether an exception is thrown or not. It’s typically used for cleanup code, like closing connections or streams.Finalize
is a method that is called by the garbage collector before an object is collected. It’s usually overridden to clean up system resources, like closing files or network connections.
Each of these concepts plays a crucial role in Java programming, and understanding them can help you write more efficient and effective code.
Code Examples
Let’s put these concepts into practice with a couple of code examples.
Example 1: Using Java final with variables, methods, and classes
// Using final with a variable
final int MAX_SPEED = 100;
// Using final with a method
public final void showMaxSpeed() {
System.out.println("The max speed is " + MAX_SPEED);
}
// Using final with a class
public final class Vehicle {
// class code
}
JavaIn this example, we’re using the final
keyword in three different ways. First, we declare a final
variable MAX_SPEED
. This means MAX_SPEED
is a constant, and its value can’t be changed once it’s set. Next, we declare a final
method showMaxSpeed()
. This method can’t be overridden in a subclass. Finally, we declare a final
class Vehicle
. This class can’t be extended by any other class.
Example 2: Using Java finally in exception handling
try {
int result = 10 / 0; // This will throw an ArithmeticException
} catch (ArithmeticException e) {
System.out.println("Caught an exception");
} finally {
System.out.println("This will always run");
}
JavaHere, we’re demonstrating the use of the finally
block in exception handling. We have a try
block that will throw an ArithmeticException
because we’re trying to divide by zero. This exception is caught in the catch
block, where we print a message. Regardless of whether the exception was thrown and caught, the finally
block will execute. This is why we see the message “This will always run” printed out.
Example 3: Overriding the Java finalize method
class Vehicle {
// Some fields and methods
public void finalize() {
System.out.println("The Vehicle object is being garbage collected");
}
}
JavaIn this example, we’re overriding the finalize
method in the Vehicle
class. The finalize
method is called by the garbage collector before it collects an object. Here, we’re using it to print a message before the Vehicle
object is garbage collected. This could be replaced with any cleanup code necessary, such as closing files or network connections.
Conclusion
In this tutorial, we’ve explored the differences between final
, finally
, and finalize
in Java. We’ve learned that final
is a keyword that makes variables constant, methods non-overridable, and classes non-subclassable. finally
is a block of code that always executes after a try-catch
block, and finalize
is a method that the garbage collector calls before collecting objects. Understanding these concepts is crucial for mastering Java, so make sure to practice with your own code examples!
Frequently Asked Questions (FAQ)
-
What is the difference between final, finally, and finalize in Java?
final
is a keyword used to apply restrictions on class, method, and variable.finally
is a block that is used in exception handling and always executes.finalize
is a method that is called by the garbage collector before collecting objects. -
What is the difference between finally, finalize, and final?
finally
is used to execute important code such as closing connection, stream etc.,finalize
is used to perform clean up processing just before object is garbage collected andfinal
keyword is used to apply restrictions on class, method and variable. -
Why do we use the finally block in Java exception handling?
The
finally
block is used to ensure that a certain section of code always runs, regardless of whether an exception occurs or not. It’s typically used for cleanup code, like closing connections or streams. -
What would happen if an exception is thrown by the finalize method?
If an uncaught exception is thrown by the
finalize
method, the exception is ignored, and finalization of that object terminates. -
Can a final method be overridden in Java?
No, a
final
method cannot be overridden in Java. This is often used to prevent altering the implementation of a method in subclasses. -
Can a final class be inherited in Java?
No, a
final
class cannot be inherited in Java. This is often used to prevent subclassing for security or design reasons. -
Can we declare a constructor final in Java?
No, constructors cannot be declared as
final
in Java. Thefinal
keyword can only be applied to classes, methods, and variables. -
What is the purpose of the finalize() method in Java?
The
finalize()
method is called by the garbage collector before it collects an object. It’s usually overridden to clean up system resources. -
Can we force the garbage collector to run in Java?
While you can suggest that the garbage collector runs using
System.gc()
, it’s ultimately up to the JVM to decide when to run the garbage collector. -
How does the finally block differ from the finalize method in Java?
The
finally
block is part of exception handling and always executes after atry-catch
block, regardless of whether an exception was thrown. Thefinalize
method is called by the garbage collector before it collects an object.
Related Tutorials
- Understanding Java’s Garbage Collection
- Deep Dive into Java’s Exception Handling
- Mastering Java’s Class and Object Concepts
- Exploring Java’s Access Modifiers: Private, Public, and Protected
- Understanding and Implementing Java’s Abstract Classes and Interfaces
That’s it for this tutorial! We hope you found it helpful. Happy coding!