JavaScript Syntax: A Fun, Free and Easy Tutorial
Hello there, future JavaScript maestro! Ready to dive into the world of JavaScript syntax? Don’t worry, we’ll take it step by step. By the end of this tutorial, you’ll be able to write JavaScript like a pro. Let’s get started!
Table of Contents
Introduction
JavaScript, the language of the web, is everywhere. It’s in your browser, your phone, and even your toaster (well, maybe not yet, but who knows what the future holds?). Understanding JavaScript syntax is like learning the grammar of a new language. It’s the foundation that allows you to write, read, and understand JavaScript code. So, buckle up, and let’s get started!
JavaScript Variables
Variables in JavaScript are like containers that hold values. You can think of them as little storage boxes with labels. Here’s how you create (or declare) them:
var x;
let y;
JavaScriptAnd here’s how you use them:
x = 5;
y = 6;
let z = x + y; // z now holds the value 11
console.log(z);
JavaScriptJavaScript Values
In JavaScript, we have two types of values: fixed values and variable values. Fixed values are also known as literals, and variable values are, well, variables. Here’s an example:
10.50 // This is a number literal
"John Doe" // This is a string literal
let x = 5; // x is a variable
JavaScriptJavaScript Operators
Operators in JavaScript are used to perform operations on variables and values. Think of them as the verbs of JavaScript. Here’s an example:
let x = 5;
let y = 6;
let z = x + y; // z now holds the value 11
console.log(z);
JavaScriptJavaScript Expressions
Expressions in JavaScript are combinations of values, variables, and operators that compute to a single value. It’s like a mini equation. Here’s an example:
let x = 5;
let y = x * 10; // y now holds the value 50
console.log(y);
JavaScriptJavaScript Keywords
Keywords in JavaScript are reserved words that tell the browser to do something. For example, let
and var
are keywords that tell the browser to create a variable.
let x = 5; // using the let keyword to create a variable
var y = 6; // using the var keyword to create a variable
console.log(x);
console.log(y);
JavaScriptJavaScript Comments
Comments in JavaScript are used to explain what your code does and are ignored by the browser. It’s like leaving a note for yourself or other developers.
let x = 5; // This is a comment
console.log(x);
JavaScriptJavaScript Identifiers
Identifiers in JavaScript are names. You can name variables, keywords, and functions. There are some rules, though. Identifiers must start with a letter, a dollar sign ($), or an underscore (_). They can’t start with a number.
let firstName; // firstName is an identifier
JavaScriptJavaScript Case Sensitivity
In JavaScript, lastname
and lastName
are two different variables. It’s like “apple” and “Apple” – they’re not the same, right?
let lastName, lastname;
lastName = "Doe";
lastname = "Peterson";
JavaScriptJavaScript and Camel Case
JavaScript developers often use camel case to name their variables. It starts with a lowercase letter, and each new word starts with an uppercase letter, like a hump on a camel’s back.
let firstName, lastName, masterCard, interCity;
JavaScriptJavaScript Syntax Examples
Let’s put it all together with some complete JavaScript code examples.
// Example 1
let firstName = "John"; // Using the let keyword to declare a variable
let lastName = "Doe"; // Another variable declaration
let fullName = firstName + " " + lastName; // Using the + operator to concatenate strings
console.log(fullName); // This will output: John Doe
// Example 2
let x = 5; // Declare a variable and assign a value
let y = 10; // Declare another variable and assign a value
let z = x + y; // Use the + operator to add values
console.log(z); // This will output: 15
JavaScriptRun Example Code in Visual Studio Code
If you run the above JavaScript example code in Visual Studio Code, it will look as follows:
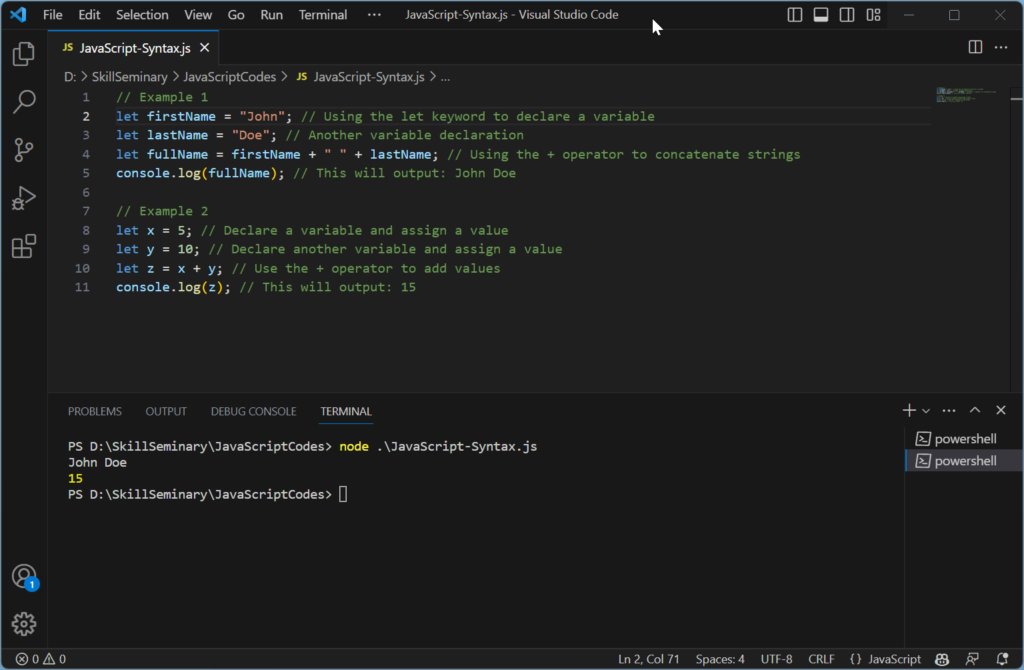
Wrapping Up
Phew! That was a lot, wasn’t it? But you made it! Now you have a solid understanding of JavaScript syntax. Remember, practice makes perfect. So, don’t forget to get your hands dirty and write some code!
Frequently Asked Questions (FAQ)
-
What is JavaScript syntax?
JavaScript syntax is a set of rules for how JavaScript programs are constructed. It includes how to declare variables, how to assign values, how to use operators, and more.
-
How do I declare a variable in JavaScript?
In JavaScript, you can declare a variable using the
var
,let
, orconst
keywords. For example,let x;
orvar y;
orconst z;
. -
What are literals in JavaScript?
Literals in JavaScript are fixed values that are not variables. They are the actual data. This can be strings, numbers, booleans (true or false), objects, arrays, null, or undefined.
-
How do I use operators in JavaScript?
Operators are used to perform operations on variables and values. For example,
let x = 5 + 6;
Here,+
is an operator. -
What is an expression in JavaScript?
An expression is a combination of values, variables, and operators, which computes to a value. For example,
5 * 10
is an expression that evaluates to 50. -
What are keywords in JavaScript?
Keywords in JavaScript are reserved words that tell the browser to perform a certain action. For example,
let
,var
,function
,return
are all keywords in JavaScript. -
How do I write comments in JavaScript?
Comments in JavaScript are written by using
//
for single-line comments, and/* */
for multi-line comments. For example,// This is a comment
. -
What are identifiers in JavaScript?
Identifiers are names. In JavaScript, identifiers are used to name variables, functions, or labels. They must start with a letter, a dollar sign ($), or an underscore (_).
-
Is JavaScript case sensitive?
Yes, JavaScript is case sensitive. This means
lastname
andlastName
are two different variables. -
What is camel case in JavaScript?
Camel case in JavaScript is a naming convention where the first letter of the identifier is lowercase, and the first letter of each subsequent concatenated word is uppercase. For example,
myFirstVariable
.
Related Tutorials
- Introduction to JavaScript
- Getting Started
- JavaScript Comments
- JavaScript Variables
- JavaScript var Explained
- JavaScript Let
- JavaScript const
- JavaScript Data Types
- JavaScript Interactions: alert, prompt, and confirm
Remember, the journey of a thousand miles begins with a single step. Keep learning, keep coding, and you’ll be a JavaScript wizard in no time! Happy coding!